Disclaimer: This post is not about security.
Is anyone else bothered by Information titled with the word security? Let me explain what it is I am referring to. I will see an article, a blog post, or a section on a web site which claims to be about "security." I will of course be quite interested when I see this, because I think security is very important when programming. One must be careful to not leave easy ways for people to exploit one's code. I think this is an important issue and it tends to be overlooked by many software writers.
The problem is that most of the time the writing I find about "security" is in actuality information about "authentication." I understand that "security" is a word which certainly can be used to describe authentication, roles, permissions, etc. I just dislike it when it is used in such a way when talking to programmers. Since it is the best word to describe the type of security which is preventing people from exploiting the code. Preventing attacks on a system is a very important aspect of programming, and it seems to be overlooked on the Internet.
I wish people would stop titling articles, book chapters, and whatever else as "security" when it is really just "authentication."
Anyone else run into any similar situations?
I recently needed to enable Mixed Mode Authentication on a SQL Server instance, so I will demonstrate how to go about setting this up. It is a pretty easy process, but I figure I'll document it nicely here. Perhaps I will come back to this later to reference this. Who knows?
First you will need to connect to the SQL Server instance using SQL Server Management Studio.

Once you've connected to the SQL Server you will need to get to the properties window for the server. To do this you can right click on the SQL Server in the Object Explorer window and it will bring up a context menu.
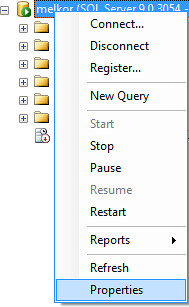
Now that you've got the properties window open, select the Security section and choose the SQL Server and Windows Authentication Mode radio button.
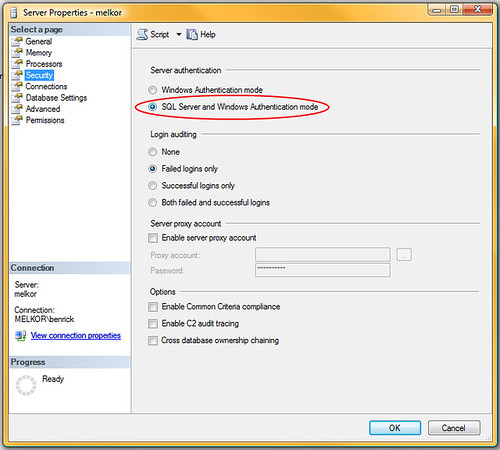
After clicking OK, you will receive a message informing you of the need to restart the Server before the changes take affect.
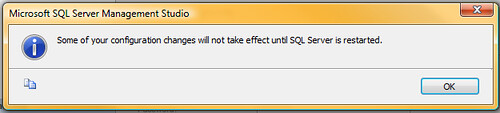
Now just right click on the server in the object explorer again and select restart from the context menu and the services for the server will restart.
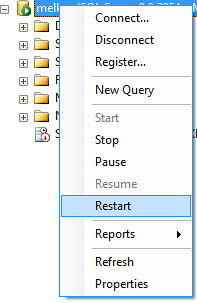
Congratulations you're done.
While installing SQL Server 2005 recently I ran into some difficulty. I use database projects from Visual Studio 2005 Team Edition for Database Professionals. I use Visual Studio 2008 with them now, and I recently made a mistake while using one of these projects. When I ran this project my default instance of SQL Server was not listed, so I figured I would connect it to the SQL Express. BIG MISTAKE. After installing SQL Server and getting it working correctly I removed SQL Server Express, and now the real fun begins. The database project no longer loads.
So I try removing and adding the project in my solution figuring it might prompt me for the database instance again. No luck. So I decide to ask around a bit. One forum got me a nice response. It explained how to make the change as well as linking to this MSDN article.
http://msdn2.microsoft.com/en-us/library/aa833159(VS.80).aspx
To make this change you just have to alter some settings in Visual Studio. Start by navigating to the Tools menu and choose Options.
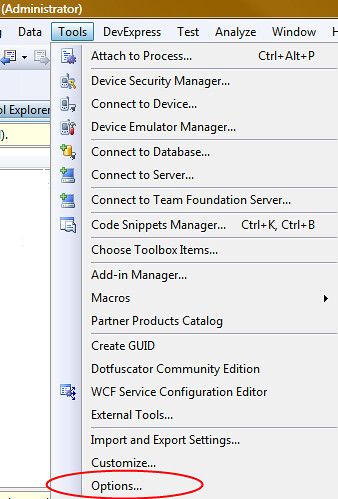
Once you've opened the Options menu, navigate to the Database Tools section and pick Design-time Validation Database. This is the information for the database used by Visual Studio for database projects. Change the SQL Server Instance Name to whatever the name of the local database is.
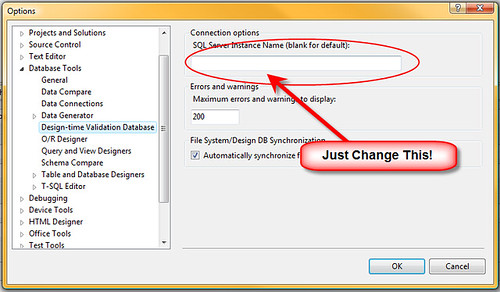
I made the bad assumption of assuming that the project is what connects to the server instead of Visual Studio. I checked everywhere in settings for the project, but it slipped my mind that Visual Studio would be what would contain that setting.
As one final note I will say Googling sucks when you're looking for information about "Visual Studio 2005 Team Edition for Database Professionals". Ah what a great name guys.....
So one of the most useful methods for ASP.NET development that never seems to be included in ASP.NET is a recursive find control method. This problem results from the standard FindControl method on controls only searching within that control. It only finds child controls not grandchildren or anything farther down the line. This means that anything nested within other controls is a pain to access.
This limitation of the standard FindControl is annoying and has prompted me as well as many other people to use homegrown FindControl methods to solve this problem. This is a big problem when trying to programmatically access controls within templates. So earlier today I read on a list someone having trouble with the built-in find control, and it made me thing that a recursive find control would make a great extension method since it is a method plenty of people want to see on the control class anyway. So I went and wrote this simple little extension method.
For this nifty example I'll use this as my Page class. I've nested some Panels here to make sure that a standard find control would not work.
<form id="form1" runat="server">
<div>
<asp:Panel ID="Panel1" runat="server">
<asp:Panel ID="Panel2" runat="server">
<asp:Panel ID="Panel3" runat="server">
<asp:Panel ID="Panel4" runat="server">
<asp:Panel ID="Panel5" runat="server">
<asp:Label ID="ControlToFind" runat="server" />
</asp:Panel>
</asp:Panel>
</asp:Panel>
</asp:Panel>
</asp:Panel>
</div>
</form>
Now you'll see here in the code behind I am calling my recursive find control extension method. Notice that I am checking for null afterwards. This is because if I do not find the control my method returns null, so I should check for this. Also because I am using the as statement if the control is not a label my variable will also be null.
protected void Page_Load(object sender, EventArgs e)
{
Label theLabel = form1.FindControlR("ControlToFind") as Label;
if (theLabel != null)
{
theLabel.Text = "Found it!";
}
}
Here is the code which makes this cool extension method possible.
public static class Extensions
{
/// <summary>
/// Searches recursively in this control to find a control with the name specified.
/// </summary>
/// <param name="root">The Control in which to begin searching.</param>
/// <param name="id">The ID of the control to be found.</param>
/// <returns>The control if it is found or null if it is not.</returns>
public static Control FindControlR(this Control root, string id)
{
System.Web.UI.Control controlFound;
if (root != null)
{
controlFound = root.FindControl(id);
if (controlFound != null)
{
return controlFound;
}
foreach (Control c in root.Controls)
{
controlFound = c.FindControlR(id);
if (controlFound != null)
{
return controlFound;
}
}
}
return null;
}
}
To create an extension method all I need to do is create a static method in a static class and pass as a parameter "this Type name" where Type and name are the type we wish to extend and name is a local variable name for the instance with which we wish to interact.
One thing I don't see in many examples of extension methods which I have used here is passing a parameter in the extension method. It really is this simple to pass the extra parameter.
This syntax reminds me a lot of the python language whose non-static class methods accept "this" as a parameter. It defines them as taking the instance as a parameter.
Have a great day!
So one thing I've always loved about Google is the I'm feeling lucky search feature. Most of the time I know that the top result is the one I need. Earlier today, I was googling for how to link directly to the Google results using an "I'm Feeling Lucky" search, and I found one interesting thing. I am not sure how accurate the information is, but it seems that Google loses $110 million per year because of the "I'm feeling lucky" button. Clicking the button means you see no ads from Google, so Google effectively does not get any money from a user who clicks that button.
OK, so by now I would hope that someone is wondering why I would want to create a link which points to an "I'm Feeling Lucky" result. Well it is quite obvious that I want to hide the eventual destination of the link. I am assuming that people will notice that it goes to Google, but will not notice the part of the query string where it really doesn't send them to Google.
So I found out that all you have to add into the querystring is btnI=745. Yes, it is that easy, so Here is a nice example of a link I believe you should all see.
http://www.google.com/search?hl=en&q=french+military+victories&btnI=745

Following the link I have listed above is the same as doing what my screen shot illustrates.
Yes, I think it is a very funny joke. That site has been the top result on Google for that search for a looong time. It is the top result for "french military victories", and is quite a funny result. It could use an update to look like the current version of the Google results page, but it is still pretty nice. I could also link to this site, and send people through Google to get there.
Orcs Goblins and .NET
Have a great day, and don't use this for anything nefarious!