
With some recent efforts from an excellent designer named Craig Palenshus, everyone's favorite regular expression library has received a nice visual upgrade. You'll notice the site is much cleaner and more presentable now. The overall design is excellent IMHO. The site seems to be much easier to use and navigate. The links are more clearly defined and laid out. The page is not quite so cluttered. In the past the site had been added to and altered plenty of times, and this created layers of clutter and bad layouts. Steve Smith and I have taken some time to fix some of the bugs from the previous system as well as getting some of Craig's alterations functioning well.
A long time ago on web site far, far away there was a regular expression tester that worked pretty well. Since then the client side tester broke, but no one really had the time to fix it. With the advent of the new look for the site soon coming, it was important to make sure that all aspects were functioning within the operational standards. One man, Brendan Enrick, set out on an adventure to complete the regular expression tester. It received an overhaul from its previous design. It not only works as it previously did, but the client side testing seems to functioning once again within normal parameters.
Ok so in all seriousness, I fixed up the regular expression tester on RegExLib. Let me know if you find any bugs or problems with it, and I'll see what I can do to clean them up. I hope you enjoy the new layout of that page. It worked pretty well for me. I've used it a bunch of times since I updated it. I find it a lot easier to use. I hope you feel the same way.
RegExLib has come a long way since Steve Smith first created the site in ASP.NET 1.0, and it has seen a couple of big layout changes. The first incarnation of RegExLib was red. The site has been green for the past few years, and it is staying that way for its new look.
This is how the site looked near its first incarnation. This is the site back in 2003 when it was still wide and red. It was originally part of ASPSmith. This is the first view of the library as its own web site. As you will notice it was reasonably well designed. The basic layout of the site is nice. The search results aren't very clean, but they work for their purpose.

Later the site received a new design of Thomas Johansen. That layout remained for plenty of years, and it received many minor changes over the years of use. This is the nice green layout everyone has been seeing for years.
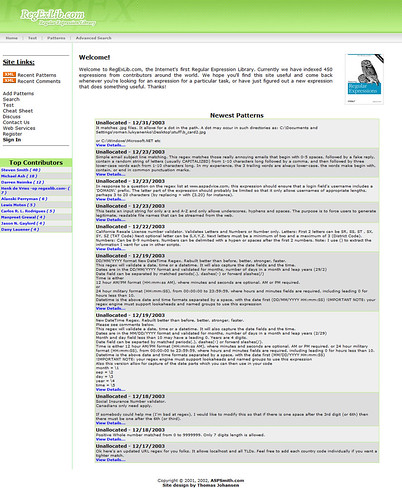
Now after trimming the fat off of the site, it is a tall, lean page with plenty to offer its users. Sections of the pages are grouped together nicely with headings. There is spacing to allow users to clearly see and use elements on the page. The RSS feed is being powered by FeedBurner so you can now see how many others are subscribing to the regular expressions on the site.
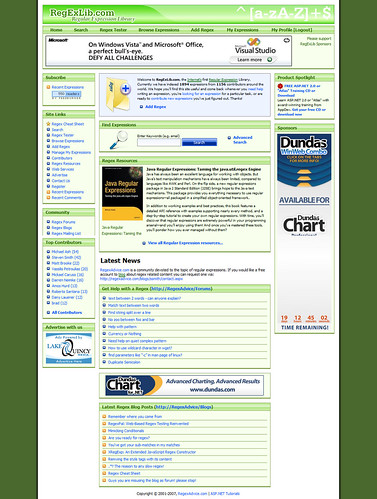
The site is more usable in my opinion. I have a much easier time reading and working with the pages on the site. I think this visual upgrade is also a very strong usability upgrade as well. I recommend you check out the new design of RegExLib.
Let us know what you think!
There are plenty of reasons why I like the Python language. Yes, I know it is an interpreted language and it is very dynamic. While looking at some JavaScript mere moments ago, I saw someone pass back two values from a function. This is one thing that bothers me when I am writing in a lot of languages. I will need to pass back two or more values from my function. So I need to change the return value to some type of a sequence perhaps an array. Then I need to adjust the location where I call the function so that it accepts and array. It then needs to take each value of the array and place the returned value into each variable that needs the value.... Yuck! What a pain just to get a couple of values!
A lot of times I just want a simple solution to my problem. I'd like to type 2 lines of code instead of 5. Python has plenty of problems, but one of my favorites assets of the Python language is the ability to Unpack tuples from functions. So lets say that I want to return three values from my function. I just separate them by commas and it works. I then in my function call just list each variable to which those values correspond separated by commas and away I go.
def GetBestHotel()
# Logic Goes Here
return name, location, cost
hotelName, hotelLocation, hotelCost = GetBestHotel()
Isn't that a neat trick. I just listed each variable and Python unpacked that tuple (basically an array) and it put each of the values in the correct variables. No extra variable declarations and I didn't have to have an assignment statement for each of the values being returned. Now that is convenience. This is obviously a very specialized little feature, but I do enjoy having it available. It is always good to know of the useful tricks of any language you use.
I recently authored an article for ASP Alliance in which I describe the basics of how interfaces work and how to use and create them in C#. I tried not to describe in great detail exactly how everything works, because that will prevent beginners from understanding how interfaces may be used. I plan to write further about this topic, but I think it a mistake to begin with even an intermediate description of a topic without at least giving a beginners explanation first. I'll be doing some more advanced examples for my next article on interfaces, so look forward to one in the not too distant future.
I am not sure how the rest of you feel, but I think that interfaces are an amazing tool. I try to use interfaces when possible, because it makes code much more maintainable.
Let me know what you think and any suggestions for future articles.
As always, thanks for reading!
It is common to obtain variables with a type which is not the desired one. Considering that plenty of times there will be variables passed using the object data type, it is important to be able to cast your variables into more usable types. Say for example you're passed a variable as an object. You probably want to be able to access properties or methods of this object, so you will need to cast it into another class. The simple way of doing this is to use code similar to this.
DataTable myData = (DataTable)dataSource;
This works well, but is slightly more dangerous than it needs to be. The problem I have with casting it in this way is that the variable dataSource might not be a DataTable, and if this is the case we will throw an exception. Yes, we could wrap a try-catch block around this code, but I prefer to avoid exceptions when possible instead of catching them.
If we use the as statement we will be able to achieve the same result and just need a little bit of checking for an error. This way if our code is in an exceptional case we can handle it ourselves instead of having the overhead of a try-catch block. If the as statement receives an invalid cast it does not throw an exception. Instead of throwing the exception it merely places the null value instead. Since we receive the null value if the cast fails, we are now able to simply check for the null value.
DataTable myData = dataSource as DataTable;
if (myData != null)
{
// Perform work here
}
else
{
// Error handling code goes here. Perhaps a message of some kind.
}
As you can see we're able to avoid our exception and handle it without using a try-catch block. I am not advocating programs without these try-catch blocks. I am merely saying that I find it better to avoid catching exceptions that could have been avoided in the first place. I think some people become excessive with try-catch blocks. I've seen plenty of people write code which doesn't check strings for validity they instead just try working with them and if an exception occurs they catch it and respond. I think it is simply crazy.
Have a great day! Happy casting!
I often write queries in SQL Server Management Studio to find different bits of information about which I am curious. This is quite easy and I discover a lot of useful and interesting things this way. You might ask why I am writing this blog post. I'll first say that I try to share these little tidbits with others. Sure I could bring people over to my computer and show them these nifty collections of data with certain meanings, but that would just be silly. I need to store it elsewhere and email it.
Maybe I'm just going about things the wrong way, but I've tried to copy and paste from SSMS into Outlook and that doesn't usually work well. Outlook doesn't seem to figure out that I would like my data pasted as a table, so I usually throw it into Excel first. Doing this will get my data into a nice tabular format.
Now that I've finally gotten my tabular data into a table everything is great. Right? No. I lose once again. I don't have the column names with my data so I end up with something like this and without column names people will not know what I am trying to tell them.
Brendan |
McAwesome |
Enrick |
5555555555 |
12345 |
9876543 |
John |
Michael |
Smith |
1234678910 |
1337 |
31337 |
So then I have to go and type out column names so that the people I send this to know what it is. Now for this example it wouldn't be too bad, but sometimes I'll be sending 10 columns worth of data and that is just annoying. I wish I could just copy out of SQL Server and have it pull column names at least then my Excel trick would at least work. If anyone knows a nice easy way to just copy out of SSMS please let me know it would make things so much easier.
Perhaps you can find some elusive option names similarly to Copy All Data With Column Names, because I sure as hell haven't found it.
In case you are wondering those column names are; FirstName, MiddleName, LastName, Phone, SomeValue, SomeOtherValue.