I have a few friends in college who are learning C# on the side. I've been answering their questions when they ask. One interesting question was regarding access modifiers. I was asked which access modifiers are used by default in certain situations when there is not one specified.
First I'll quickly remind everyone of the access modifiers and what has permission to access them.
private - Any members of the same class have access.
protected - Any members of the same class or any derived class have access.
internal - All code in the same assembly. This means that it doesn't matter where the code is in the assembly. It can be in any class as long as it is inside of the same assembly it has access.
public - EVERYTHING HAS ACCESS!
When you define a data type of some kind (class, struct, enum, etc.) you need not specify an access modifier, but be aware that the default will be internal. This means that anything else in the assembly has access to it. There is very little sense for it to be private, because that would mean that only it has access to itself which means it would never do anything. Since we like the default permission to be as restrictive as possible it makes sense that the default is internal.
The other code with access modifiers are the members of the types we were just discussing. Since these are within a type private makes sense for them, and since we like keeping this locked down by default they use the private access modifier if no other is specified. This means they are only accessible by other members of the same thing. So a class might have a private member function and that function can only be called by other members of the class; functions, properties, etc.
Protected is probably my favorite access modifier to use, but since it is less restrictive it is not the default. This is because pretty much any time protected can be used so can private so private will be the default. Public is just way to open to be the default and C# doesn't want to encourage programmers to have everything be public. That is just a bad way to write code.
Don't get locked out because you don't know the default access modifier. Make sure you key in to the correct way of writing your code.
Have fun writing code with access modifiers!
Recently I've started using CodeRush™ with Refactor!™ Pro after being defeated in a programming competition at DevConnections by Sarah (a hired model) at the DevExpress booth. There should be two recordings of my painful defeat. Steve Smith made one and Carl Franklin made the other recording of my battle. Just for the record Steve faced her days earlier when she was still learning and he also lost.
Overall I've found the tools to be extremely useful. They make a lot of tasks quick and painless. Classes, methods, and properties are extremely quick to write now. Refactoring catches me off guard sometimes, but I am often pleasantly surprised.
For simplicity I was calling a method inside of a foreach loop. I did this because I didn't need the collection except that I was performing an action on each of the returned elements in the collection. When I realized that I needed to perform an extra action with that collection I decided I would move it from the foreach loop.
At first I had a foreach loop similar to this one.
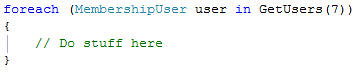
As you can see my foreach loop is using a MembershipUserCollection returned from a function and is going to loop through each MembershipUser. It is at this point I decide to refactor and pull the GetUsers method out of there and I am going to make a variable to store this collection. I highlight then cut the method and paste in in the line above and this is what I get.
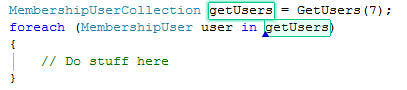
It knew what I was trying to do! It created the collection and even inserted the variable into the foreach loop for me. When I then rename the variable it also changed it in the foreach loop for me which was quite nice. I didn't even have to check and see what type of collection it was returning before creating the variable. That might have been a generic list, but it didn't matter because the tool figured it out for me and filled in the rest. I was amazed.
There is one annoyance that bugs me though. I have habits I've gotten in to while writing code. I don't like hitting ctrl+space to bring up intellisense. I prefer removing a comma or a parenthesis and typing it again. This works very well without having any extra tools installed. It also works well most of the time. I've only run into a problem a few times.
I start out with code that looks like this. There is a long function call so I keep the parameters below the function.

Now I want to get intellisense back just to confirm the first parameter or maybe to see the overloads for this function so I delete the "(" and put it back in place and I get the following.

Now it has added in an extra ")" that I did not want. I wish it could have seen that my function was complete and I was at the start. Sure that might have been convenient if my code wasn't already written, but in this instance it got annoying. Visual Studio got confused and gave me nice red squiggly lines. DOH! Not too terrible. Most of the time the tools work well there are just a few quirks like this that get me sometimes. Perhaps this blog post will help get the ball rolling to make this product smarter and better able to see what I'm doing. Overall I think the tool is excellent.
If you're interested in buying any cool software from Dev Express I recommend you check out Steve Smith's Dev Express Tech Summit blog post. He mentions a good discount code there that will get you 10%. His coupon code is SMITH07 you can use it to save 10% when you buy great programming tools from DevExpress.
Happy coding!
There are plenty of AJAX tools being used everyday. The AJAX Control Toolkit can be likened to a pack of Bertie Bott's Every Flavor Beans; there are far too many choices. Some tools you'll love and some are as useless to you as an earwax flavored bean. Likely no individual developer will find a use for every control in the toolkit, but there is one which I think has a simple use which can be applied to nearly all sites; the TextBoxWatermark control.
When using the TextBoxWatermark control a developer is able to easily place text inside of a TextBox for a user to see. In my experience I've noticed that users tend to read as little text as they can when using forms. It is also difficult sometimes to write necessary information within strict size constraints. Plenty of text boxes require a title on the left so a user know what to type in the box, and then there might be another message below giving more specific instructions.
My personal favorite method for using this control is to place the title of the TextBox to the left and then placing an example in the TextBox as a watermark. This gives the user a nice example which cannot be easily ignored. Overall a very useful control. It is also very simple to use.
<ajaxToolkit:TextBoxWatermarkExtender ID="TextBoxWatermarkExtender1" runat="server"
TargetControlID="TextBox1"
WatermarkText="YourDomainHere.com"
WatermarkCssClass="watermark" />
<asp:TextBox ID="TextBox1" runat="server" />

Using this control it is simple and easy to pass along great information to the user which he or she cannot ignore. It can also make a form look more professional. I am not saying that the TextBoxWatermark is the only useful control. Most of the tools are very useful, but some have some obscure uses. Some of them I know when people use them I just think they shouldn't use them.
Happy AJAXing.
Earlier today I downloaded IronPython, and I've tested it out a little bit. The command line interpreter is nice and works pretty painlessly whether you are compiling the source or just executing the binaries. Python is a great language IMHO. Just like every language that has ever been written, it has a few problems. Python emphasizes short readable code.
IronPython's interpreter, ipy, allows interactive sessions as well as execution of files. It acts very similarly to Python's Official interpreter, IDLE. This makes transitioning easy for someone who already knows how to program using the Python language.
I sat down and took a couple of seconds writing a simple fibonacci number program to print out the first 10 fibonacci numbers as an example of how Python code is used.
def fib(number):
if number == 0:
return 0
elif number == 1:
return 1
else:
return fib(number-1) + fib(number-2)
def main():
for i in range(10):
print fib(i)
main()
If this code is in the file fib.py I can execute it using the following command.
ipy fib.py
It generates the following output.
0
1
1
2
3
5
8
13
21
34
People have integrated IronPython into Visual Studio as well, and I'll soon look into doing that as well. I like how simply and easily I am able to create this little program. Now with IronPython I also have access to the .NET Framework's libraries.

In my attempt to add to the monotony, I'll say that not long ago Visual Studio 2008 was released to all of the MSDN subscribes. With the news spreading so quickly the downloads are all taking forever. Good luck! Scott Guthrie, as usual, posted some good content about this Visual Studio 2008 release. There will be some trial versions of the professional available in the not too distant future.
You can also read a nice tour of all of the new features added into this new release. Scott wrote short overviews for a lot of the information and linked to more in-depth descriptions of these features. Make sure you follow those links if you're not well informed about this release.
The Visual Studio Express Editions and the .NET Framework 3.5 runtime are currently available for download. These downloads should work better than the MSDN full version of Visual Studio. These are not multiple gigabyte files and also are probably not as popular right now.
I've been hearing complaints all day about difficulties trying to download it. I am assuming the problems result from too many users attempting to download. Good luck and enjoy some of the great new features.