Some very surprising news has come from Scott Guthrie in a recent blog post he has made. Visual Studio 2008 and .NET 3.5 will be shipping with the source for the base class libraries. As with a lot of the recent technology coming from Microsoft, the source code is open and not hidden away.
In the past, tools like Reflector have allowed developers to see what is going on within those libraries. These tools will no longer be as necessary. This source code is being released under the Microsoft Reference License (MS-RL). It seems Microsoft has started a new trend for itself in releasing its source code.
Debugging will now become much much easier with this code being open source. In the past developers have not been able to step into any of the base class libraries. Now that the source is open for everyone to see, people are able to go in and step through this code. This should make debugging much easier and better.
I think this is great that Microsoft is releasing more source code to the general public.
Happy coding.
Outside of SQL it would be quite easy to number the rows returned from query, but to do so inside of the SQL is a bit more difficult. I recently wanted to be able to number the rows returned from a query in a stored procedure. I am using SQL Server 2000, so I cannot use row_number().
I was going to ask my go to SQL guy, Gregg Stark, how to do this, but while in the process of asking him for a good method of doing this, I came up with the solution. My cool trick for numbering the rows returned from a query is useful because I don't actually want to return these rows to other code. I want to store them back into my database ordered differently than the numbering, and using a table variable will allow me to do exactly what I am trying to do.
First we need to create a table variable. We will define that table as having an identity column plus any other columns we happen to need. We then insert into that table all of the values returned from our query.
declare @t table (Rank int identity(1,1), UserName nvarchar(256), Points int)
insert into @t
select UserName, sum(PointsEarned) as Points
from UserRecognition
group by UserName
After running this part we have created a table variable which contains the information we want. We can then select data out of this as if it were a normal table. In this example I am able to obtain a ranking for my users based on their earned points. This lets me store that ranking without having them stored in ranking order.
It is obviously much easier to do this in SQL 2005, but this is a very cool trick for those of us using SQL Server 2000. Let me know what you think, and if you have any cool improvements.
An interesting question came up from a few of my students while teaching my class. Some of them discovered a bug in their programs which they did not understand. They were writing a program which was doing some calculations on currency.
Computer Programmers commonly use floating point numbers, but I am sure that not all of them really understand what is going on underneath the hood. As I am sure everyone reading this knows, all data is stored in binary format on computers. In base 10 as with all numbering systems with a base, each digit is a round number in the base. The fractional amounts are what is interesting and specific to floating point numbers and not to integers.
When using floating point numbers they aren't stored in a way which is intuitive to base 10 users. As an example I will use the fractional amount 1/4. To make seeing the conversion easier I'll say that it is also 25/100 or really 2/10 + 5/100.
In a base 10 floating point number we may represent this number as the following.
0.25
If we were to write that same number in binary format we should note that it is 1/4 or really 0/2 + 1/4.
0.01
You'll want to notice here that those two numbers are not similar, and as you can probably guess, some examples would not come out quite so cleanly.
The problem which the students came across stemmed from subtracting 10 cents from some amount of money. I'll use $100.00 as my example. When the students did the calculation it worked in the following way:
100.00 - 0.1 = 99.900000000000006
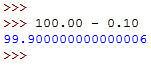
That seems to not make sense, but it does make some sense. There are numbers which in base 10 will have decimal places which will extend on, and we have to round them at some point. A good example of this is 1/3 which can be written roughly as any of the following
0.3
0.33
0.33333333333
All of those are approximations of that fractional amount. In binary we have the same type of problem, because 0.1 cannot be represented correctly in binary. This causes a problem; it will round at a certain point, and because the number isn't represented correctly, the calculation is done incorrectly. In many other languages it hides away that inaccuracy, but Python allows you to see it.
It is important to know how your floating point numbers are working underneath. Be careful. The computer doesn't speak our language.
I recently started teaching a course for the Computer Science Department at Kent State University. I am teaching a course called Introduction to Computer Programming. It is a course teaching non-computer science majors the basics of programming.
In this course the students learn simple concepts and ideas behind programming, and along the way will learn to develop simple applications. The students who take this course come from many different areas: Technology, Education, Art History, Visual Communication Design, etc.
I am enjoying teaching the course, and it is beneficial not just to the students but to me as well. As I am teaching this course I am refreshing my memory on a lot of different areas of computer science which I believe are very important to software development.
Python is the programming language of choice for this class. It has been chosen for many reasons, but a few choice ones are: Python's being a very high level language, ease of use, quick development, works with Windows, Linux, and Mac OS. We have Mac, Linux, and Windows users in the class so it is nice to use a language which is easy to develop in and works well in each of the operating systems.
In an effort to start posting more to my blog perhaps I can post a few of the interesting concepts which are applicable to all programming not just Python.
Update (24 March 2008): Steve Smith found a more reliable solution to install the SQL Server Client Tools. Once in the tools folder open the setup folder and in there is a SqlRun_Tools.msi file. If you run that it should actually install SSMS.
When I recently installed SQL Server 2005 and SQL Server Management Studio on my computer it did not install SSMS or any of the other Client Tools. When running the installation of SQL Server 2005 I followed along with the instructions. I individually selected each component to install including the Client Tools for SQL Server. When the installation finished there was no SQL Server Management Studio.
Figuring this might be a difficult thing to Google for, I asked Steve Smith if he knew how to get the client tools. He told me that just about the only way to get SSMS to install was to sacrifice a chicken and hope for luck, because there is something weird which has to be done in order to get the program to install.
Upon scouring the folders on the disc, I discovered that the default setup file is coming from a servers folder. I tried using the setup file from the tools folder. This should have worked, but I had a slight problem. Since I had told SQL Sever to install the client tools from the wrong setup file it now believed I had them installed already and would not install them.
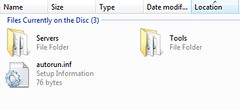
Since the installation defaults to the Servers setup file, I never even saw the tools install. There was also no reason to even suspect one since the primary setup file claimed to be able to install the client tools.
I had to uninstall and reinstall SQL Server without the Client tools and then the setup file from the client tools would install SQL Server Management Studio. This was quite a pain, and I don't understand why the client tools are listed as options in a setup file which cannot install them. I think this is a bit crazy, but at least now when I install it I don't have this problem anymore.