This is the first post in a series that I am writing that focuses on some interesting finds from different code bases I’ve seen over my years looking at lots of different software projects. Code Audits are one place where lots of interesting bits of code can be found, since you're combing through an entire software system. I will be explaining what I’ve seen, why it’s wrong, and usually the better approach if it’s not obvious.
URL Parameters
URLs let you control the navigation as your user navigates around your site. Users may not know what URLs mean or how they work, but most people browsing the web know that URLs exist. A good number of them know that modifying that URL will take them to different pages. Even my mother knows that much about URLs.
It’s not just a tech savvy computer programmer or hacker who can figure out that changing the URL might get them to a page they’re trying to see. This is sometimes useful on site’s whose navigation is somewhat lacking. It’s also often possible to guess the page you’re looking for by adding “/login” or some other common word to find a page whose link you’re not seeing.
The Bad
On web sites that have user accounts associated with multiple accounts, it’s quite common for the current account that the user is viewing to be specified in the address bar using a query string parameter. Normally, this means that there will be something along the lines of “?AccountId=123456” on the end of the URL. It’s also very common for those to be built in as “subfolders” like this “/Account/123456/”. Either way, it’s easily visible, modifiable information presented directly to the user.
As I am sure most of you can predict, I am going to point out that I’ve seen some code that didn’t do the proper protection to make sure that only an allowed user can access any given AccountId. The site will only display the links to the allowed accounts, but the site itself does not protect against a user changing that AccountId.
If you want to get to another user’s account, you can just type in another AccountId into the URL and the site will take you there…
The circumstances where these mostly come up is when 1 user account is allowed to have access to more than one account. It’s on these sites that the user will need to be able to change accounts, and sometimes users keep multiple accounts open at the same time, so the URL is needed to keep both open at the same time. It’s important, however, that we maintain at least some level of security here.
The Better
When we build sites, it’s extremely important that we validate inputs given by users to make sure that they’re valid. It’s also important to make sure that users have access to the information they’re requesting.
The first thing you do when you receive user input is to validate that input. Obviously if you consider a URL to be user input, you would validate that immediately as well. This means that either directly or through some layer of abstraction, your code should be verifying a user has access to any information being requested.
Citigroup
There was a big fiasco last year related to Citigroup. (http://www.nytimes.com/2011/06/14/technology/14security.html) The rumor is that they had a security breach based on this type of issue. I have no real, credible information about this, but it makes for a good example even if it’s only rumor.
From the New York Times article we get these:
Once inside, they leapfrogged between the accounts of different Citi customers by inserting vari-ous account numbers into a string of text located in the browser’s address bar.
That sure sounds to me like what I just described. If it is what I described, then this security expert stretched the truth quite a bit based on what is in that same article.
One security expert familiar with the investigation wondered how the hackers could have known to breach security by focusing on the vulnerability in the browser. “It would have been hard to prepare for this type of vulnerability,” he said. The security expert insisted on anonymity because the inquiry was at an early stage.
It’s not hard to prepare for that kind of vulnerability. I guess if you have it, it’s hard to track and log the issue. What’s easy is not having the issue to begin with. It’s a simple policy of making sure that all inputs are validated for permissions before being executed. Also, that is not a “vulnerability in the browser”.
More Code Audit Nuggets
Keep watching for more interesting nuggets of stuff that I’ve seen in codebases.
Now that we’re in the second half of June, I think it’s time that those of us with one, take a look our 2011 Software Craftsmanship Calendar. In June 2011, the topic was the Single Responsibility Principle, which states that your code should only have one reason to change.
The Single Responsibility Principle is the first in a set of Principles called SOLID,
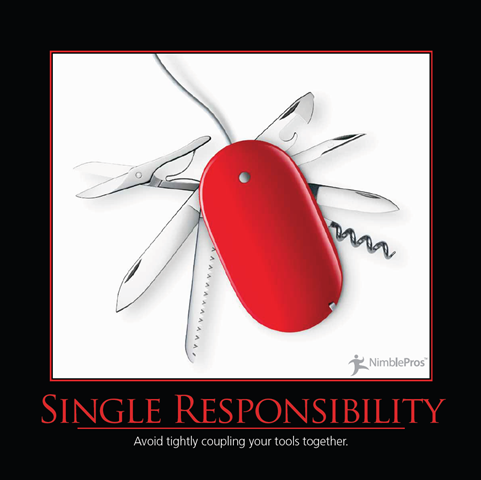
That looks dangerous…
For the rest of June, please try to follow Single Responsibility Principle and try to not be a Duct Tape Coder. Everyone who has to maintain your code in the future will thank you.
For anyone following along in the NimblePros Software Craftsmanship Anti-patterns Calendar, June is the month of the Duct Tape Coder. The calendar is a great reminder to try to avoid all of these bad practices. You obviously want to avoid all of the bad practices illustrated in the calendar, but for the month of June, you should be focusing on not being a Duct Tape Coder.
Duct Tape Coding
Similar to Cowboy Coding, Duct Tape Coding is all about getting things done. Not worrying about how you do it or how it will be maintained in the future. The goal of the duct tape coder is to get something working.
You might be saying to yourself, “I thought that all of these agile good practices said to start with the simplest thing that works!”
You’re correct. It says to start there. That means that you need to clean things up and use refactoring to create a maintainable, clean solution. Consider the initial duct tape to be scaffolding to allow you to build the final, maintainable solution. The duct tape, along with some automated tests allows you to build a system to be proud of.
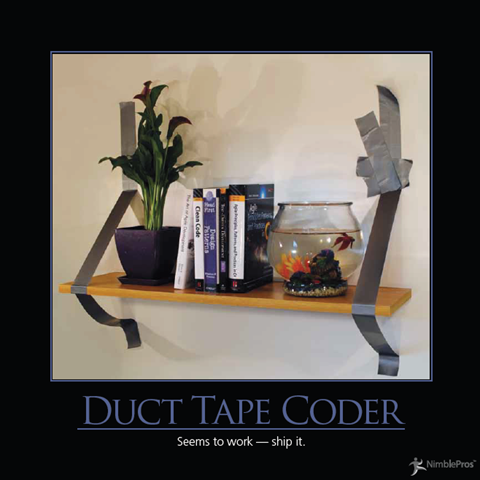
Spend June making sure that you’re building your software the right way. Don’t settle for a “working” solution. Build a good solution.
I recently read The Clean Coder: A Code of Conduct for Professional Programmers (Robert C. Martin Series)
, and it is one of the best books on software development that I’ve ever read. The book is a quick read from Uncle Bob Martin and is comparable in size to his similarly named, Clean Code: A Handbook of Agile Software Craftsmanship
.
The book goes through many, many examples from the author’s development experiences. It has sample discussions between parties to explain points and really goes over professionalism in software development.
The Title
The impression I have about why the book is called “The Clean Coder” is mostly a play on professional developers wanting to have clean code. This doesn’t just mean the code, the code is included of course, but it also means that the whole coding experience and the whole project should be clean.
There are a lot of mistakes that developers can make, and the book is covering them and discussing how to keep things clean.
The Material
Without jumping into too much of the meat of the book, I will say that there are many things in the book that I’ve done before and don’t do anymore. There were some things mentioned that I’ve done recently, and I am going to make sure I never do again. There are some things in there that I’ve thankfully never done, and I hope I don’t ever do them.
The book focuses on a lot of bad situations, why these situations are bad, and how we should really be handling things.
Consider this book to be a guidebook of suggestions for how to act as a professional. For example, in the book’s discussion of estimation, instead of focusing on how to be more accurate with estimation, it covers how you should be estimating. This means that it tells you that you should be very clear about things being estimates, not to imply a commitment, and not to make commitments if you cannot meet them.
Controversial Points
The Clean Coder makes some recommendations that I am sure that plenty of people will disagree with, and the way some points are worded cause me to sometimes disagree as well, but I don’t think it really takes away from the value of the book.
One example of this type of suggestion is that you work 40 hours a week and then another 20 each week on improving your career, leaving 56 hours for sleeping and 52 hours for everything else. It’s presented as doing this makes you a professional and not doing it is not professional. What I disagree with is the definite amount of time. I think it’s important for people to work on their careers, but 20 hours is arbitrary here. Someone may get the same value out of 10 hours that requires me 30 hours. There also is no definitive line between professionalism and unprofessionalism. There are some gray areas, and I believe this would be one of them.
Some things in the book were kept clear that it’s how he works, and I took these as suggestions to learn how I work and make sure that I am evaluating a possible issue.
In this case, I am referring to a point on music. Some people listen to music lightly while programming. This is even true when pair programming. I sometimes like having a very light bit of background buzz from music while pairing. In this case, I keep it so quiet that we can still communicate easily. The book seems to be against music, since it distracts and changes the author’s thinking. For me, I think I don’t have this issue because my pairing partner prevents a change in thinking. I also try to listen to music with foreign lyrics or no lyrics at all. It also helps to not distract from the task at hand. The value here for me is that I should be evaluating and thinking about how I am doing things to make sure that something as seemingly inconsequential as music is not detracting from my work.
Meetings
One great suggestion made by Uncle Bob is that it’s OK to decline a meeting invite. I think that’s very important to understand. Unless you’re really needed in the meeting, you should try not to attend. Meetings take up time, and if it’s less important than your other work, you shouldn’t be attending.
I had a meeting I scheduled yesterday. I invited 8 people to that meeting. I made sure it was clear that if you had something to bring up, you should attend the meeting, otherwise your attendance was not required. We had 4 people in the meeting, and I think 1 of the attendees need not have been there anyway.
Trying
You should read the section on trying. It’s important not to try, and this book makes it very clear why. If you’re going to try, you’re making a commitment to try.
Mentoring
The first paragraph on the chapter on mentoring illustrates one of the biggest shortfalls of the software development industry. I owe a lot of my successes as a developer to the mentoring that I’ve received through books, blogs, and any other recorded sources of information I can get my hands on. I was also lucky enough to have a mentor and a role model to learn from as I became a better developer.
Summary
If you go in to this book expecting it to be a recipe for success, you will be mistaken. This book is fantastic, but it’s important to think and consider why it’s valuable. It is one developer’s experience as a developer.
From his own stories, he is a developer who has hit some pretty rough patches and made some terrible mistakes. That’s a good thing! It means that he has learned a lot!
While reading the book, I never felt like it was forcing his way of doing things onto me. It is presented in a great way to inspire changes in the developers who read the book. You don’t have to do the same things that he is doing, but try to consider what the right answer should be.
The examples are simplified for the book, and business are more complex than what is in the examples. The reader will need to adjust and make decisions, but that’s what you should be doing anyway. Think about being professional and doing what you should be doing.
This book has been on my intended reading list for a while, and now I am going to recommend it to other people.
Well done, Uncle Bob. Thank you for sharing your experience with everyone.
After CodeMash, I was informed by many people that I had missed a great talk that Scott Hanselman presented. The talk was his “Information Overload” talk, which is about the onslaught of information that the world sends at us constantly. I’ve since discovered that he has been presenting this around the world. Last week, at StirTrek, I made a point of watching Scott’s “Information Overload” talk.
His talk is designed for developers, but it’s not developer-specific. It is all about managing the streams of information that come into our lives. As is usual, his talk is entertaining, well-presented, and contains enough impromptu comments and laughter to keep it memorable. (More than just the comedy is memorable even.)
He had a lot of suggestions about how to organize what is important, when we should deal with things, and how we should deal with things. Techniques, tools, recommendations, and guidelines are the name of the game.
If you get the chance to see the talk in person, I recommend doing so. It is one of the better talks I’ve seen presented at conferences.