When I say "Try Methods", I am of course referring to the common prefix "Try" on a method, which implies that the method is going to attempt to do what you're asking by using an output parameter for the operation and using the return value to indicate whether the attempt succeeded.
The common ones that people see in the .NET Framework are the TryParse methods, which attempt to parse something and if it can't be parse, they assign the default value to the output parameter and return false. If the succeed, the parsed value will be placed in the output parameter and the return value will be true. If the code failed, the convention is to use the type’s default value to assign to the output parameter. This lets you write code like this:
int someNumber;
int.TryParse(userInput, out someNumber);
// use the number entered or the default 0
int result = 1 + someNumber;
This is also great when you're going to be checking whether the method succeeded. In fact, you can use the try method directly in the if block, which usually makes things nice and clean. Here is an example of using them like that:
int someNumber;
if (int.TryParse(userInput, out someNumber))
{
// Do something with someNumber
}
else
{
// Invalid input: handle this case accordingly
}
Yes, you know all of this and have seen it before, however, are you writing these types of methods yourself or is your only experience with them the standard TryParse ones?
What I am trying to emphasize here is that you need to write your own Try methods in your code. You will thank yourself later.
Everyone can see the obvious benefit of not having to check for the default value to see if it the method worked. You also don't have to have ugly Try-Catch logic in your code. You get an if statement instead.
The real benefit of a writing a Try method has nothing to do with what I've already said. The most important benefit, in my opinion, is that you have a return value from it that isn't the value you are using for your logic. By having this return value for its success, you have to decide on how to handle the case where it failed.
There are important cases that might be forgotten, but having a return value means that I need to handle the return value. When I have it, I need to decide what to do with it. There may be a message I need to display to a user. There may be logging I need to do. I might be able to ignore that case. What is important, however, is that I thought about how to handle it. I had the chance to make sure that I handled the case correctly.
Create your own “Try” methods. I recommend looking into creating your own “TryParse”, “TryGet”, TryDelete”, and anything else that has a chance of failure that you may want to handle.
Classes that are used together are packaged together.
This is the April topic from 2011 NimblePros Software Craftsmanship Calendar, which includes a nice quote.
Common Reuse Principle (CRP):
Classes should be packaged together when reusing one will mean reusing them all. Source: “Agile Principles, Patterns, and Practices in C#”
by Robert C Martin
This is a pretty simple principle, and one that should seem quite logical. Not everyone agrees with the principle, but it least has a good point. If two things are going to be reused together, it makes logical sense that you should package them together.
Imagine if we didn’t follow this for ASP.NET and instead had everything spread around in some random assortment of assemblies. If I were accessing my HttpRequest, which is normally in the System.Web assembly and doing something with the cookies from that, which is an HttpCookieCollection. That HttpCookieCollection is a collection of HttpCookie. All of these are in System.Web, since they’re commonly used together. There is one assembly that we need to use this full set of classes.
Now think of how much fun this development experience would be if we had broken these apart such that the HttpRequest was in the System.Web assembly, HttpCookieCollection was in System.Web.Collections assembly, and HttpCookie was in the System.Web.State assembly. That would mean having to access three different assemblies to get three closely related classes.
Don’t get me wrong, I like having smaller, broken up dependencies, so that I don’t have to depend on a lot of things I don’t need. In fact, I have to have a good number of assemblies when I use the onion architecture. (They’re not all compile time dependencies everywhere they’re used though.)
The painful part, however, is when there are obvious pieces that should be together and aren’t. Common reuse is basically saying that there is pretty much no time that I would use HttpCookieCollection without also needing HttpCookie, so they should be in the same package. (Just those two from my example. I think that HttpRequest could be somewhere else with a good reason for it. Although we all know that all of these classes are in the same assembly right now.)
What’s your take on it? There is a lot of gray area with Common Reuse Principle.
One of my all-time favorite movies is Groundhog Day. In the film, the main character, Phil, repeats the same day for thousands of days. I've heard rumor before that the author said that Phil had repeated the day enough times to have been trapped in the loop for 40 years. I don't know if the author really said this, but it seems like enough time to have learned and experienced everything that he claims to have experienced in the film.
I believe this comedy offers a lot more meaning that what it appears on the surface. I think that we should all be striving to live our lives as Phil did in this one. No, ignore all of the silly, crazy, and suicidal days. Focus on the days where he was focusing on improving himself. Phil did a great thing in motivating himself to learn to speak French, play the piano, and genuinely change his character and outlook on life. He attained many artistic abilities and social skills. He also learned a great deal by varying his days. He repeated the same day over, but he never really repeated the day the same as he did before.
One of the greatest weaknesses that we as humans have is our urge to do today the same things we do every day. If we don't get out of our confort zone and try new things we will never learn, grow, or master anything new. I think I should strive to live my normal life more like Phil's.
I've heard the reverse mentioned about many of the developers in our industry. On many occasions and from many people, I've heard the saying that someone may have 10 years experience, but it's really 1 year of experience repeated 10 times.
That terrifies me. I really don't want to fall into that situation. i want to make sure that I am always learning and growing as a developer. I look at my code from 5 years ago and can't believe I wrote things that way. I look at my code from 6 months ago and am also glad I've improved. Ten years from now, I hope I will still be saying the same things about my past code. I also hope that the software I am writing, the languages I am using, and the patterns I am folliwing are vastly different from the ones I am using now. That will mean that I am improving my skills as a developer and improving myself.
The best way to describe this is to say that Phil took one day and expanded it to more than 10 years of experience. As developers, we should make sure that we don't condense 10 years of experience down to 1 of fewer years.
Try new things and continue improving.
One of the coolest (and scariest) things I’ve seen lately is mocking static classes and methods with JustMock. I was working on some legacy code, and was making some changes, so I wanted to make sure that I got everything tested first. When I went to write the tests, I noticed that I needed to wrap and hide away a static method that was being called.
My eventual intent, however, is to get rid of the static class and method calls if possible. Writing the wrapper around it temporarily, so I can get tests in place before refactoring has always seemed like a pain. I went to check and see if JustMock would be able to mock a static class. That seemed like one of those crazy things that commercial mocking frameworks can usually do. I was right. It could mock the static class. (SCARY!!)
I wrote some code that looked like this: (Sorry I can’t show you my clients’ code in my blog without their permission, so you get sample code.)
public static class MyStaticClass
{
public static int DoSomeDependentStuffThatIsHardToFix()
{
// Pretend this does more than just returning 1
return 1;
}
}
In my example, I need to test some code calling this static method. Normally, I would put a wrapper around this class, write my tests, refactor, and eventually change this to not be a static dependency anymore.
I decided to try this. Here is a test class that uses mocking to demonstrate how I can decide any result for this method and have control of this dependency. Notice that my method always returns 1. Pretend that a different result were possible.
Now when I go to write my test, I can just Mock out the static and define that I want that method to be called and for it to return 2 instead of 1. I will then assert that it worked as I expected in my test. Once I confirm that the mock works as expected using this type of test, I can put it in place in my actual test. (Yes, I sometimes write temporary tests to confirm what I expect before I write the whole test. Little steps.)
[TestFixture]
public class MyTestClass
{
[Test]
public void WhatIsTestedDoesNotMatter()
{
Mock.SetupStatic(typeof(MyStaticClass));
Mock.Arrange(() => MyStaticClass.DoSomeDependentStuffThatIsHardToFix()).Returns(2);
Assert.AreEqual(2, MyStaticClass.DoSomeDependentStuffThatIsHardToFix());
}
}
Then when I go and run my tests, I get this nice result showing me that everything is working as I expect it to. Yippee!
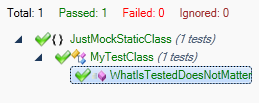
How it achieves this? I am not sure. I intend to find out though. When I do, I will write a post explaining it.
Have a good day!
This month at the Hudson Software Craftsmanship group, we had two interesting discussions that were spawned from the NimblePros Software Craftsmanship Calendar. The calendar’s topic for March 2011 was the Open/Closed Principle and the topic for March 2012 is Reinventing the Wheel. Keep in mind that 2011 is a calendar of good practices we want to encourage and the 2012 calendar is full of anti-patterns to be avoided.
This post is a recap of some of the stuff we discussed at the March, 2012 HudsonSC meeting.
Reinventing the Wheel
Going into the meeting, I had the thought that Reinventing the Wheel is obvious when you’re doing it and Open/Closed is less obvious when you’re not following it. Boy was I wrong. The main discussion we had about Reinventing the Wheel was based on “how do I avoid reinventing the wheel in a large, badly organized code base”. We had a lot of very good ideas and suggestions about how we can try to avoid this through tools, communication, and a variety of other methods and ideas. It’s interesting though, because none of them really are the silver bullet that just solves this challenge. If you don’t know if something is already written in the codebase, it can be a real challenge to find.
Open/Closed Principle
For the open closed principle, we discussed how we use it, when it’s used, and whether we should start of our code building for open/closed. I think most people came to the consensus that it’s likely not a good idea to use Open/Closed right away, but that we should refactor to it when we’re changing the code. The thought here is that if we change it once, we might need to change it again, so the next time we need to extend it instead of changing it.
Make the code better now and we’ll save ourselves time later.
Supermarket Checkout Exercise
As our programming exercise at the end of the day, we decided to do the Supermarket Checkout programming exercise. It is an interesting little challenge that involves some design decisions. This is what makes it interesting.
The exercise has the developers writing a checkout system for a supermarket. Essentially, it’s about calculating the price of the items as their scanned. It factors in discounts, selling by quantity or individually, and selling by weight. This information also needs to be presented to the customer on a receipt at the end of the transaction. This means that you need to figure out how to give the user the needed information in a nice, clean way.
As you work on the code, you have to make some decisions on how the code is going to interact with the customer. Should the discounts appear inline immediately or at the end? Is the discount a separate line item or shown in the price? How do these choices affect how you’re writing the code? Some have you returning information immediately and some have you gathering the information later. How do you adjust your design decisions based on this?
You can find a link to this programming exercise and more on my List of Katas and Programming Exercises. And, because I am not a total jerk, I will give you a direct link to the kata pdf in case you don’t want to check out my other blog post.
Supermarket Pricing Coding Exercise (Sample Output)
The Sample Output is optional to use. You can also come up with any sample output that you wish to use for it. Change things up. Or do the kata and then pretend that the business rules have changed and now you need to display the information differently.