The cloud is nice and useful, but there is one feature which is vitally important to most applications in my opinion. The storage which is used in Azure pretty much will need to be used by every application. One of the reasons for this is because there is a little bit of management of processes which needs to be done. This I've noticed requires a combination of the Queues and the Tables.
Queues are great because roles are able to queue up work which needs to be done. This helps make sure that all required tasks can be completed. Without these communication between the processes could get a bit messy. Also the information being worked with needs to be stored in a shared location which all of the roles and their instances have access to. The storage makes this work.
For these apps you don't use the standard configuration files to store your config settings. There is a file in the Cloud project called ServiceConfiguration.csfg, and this file is where the ConfigurationSection is located. This is where you define the settings for connecting to your storage when it is in the cloud. You will also have your configuration information stored in your web.config, and this will make things nice and easy. Since there are methods to get your configuration information which know to check the correct location.
For now these connections are made using http, so these "endpoints" are simply URLs. For local work the dev fabric and the storage are local. Because of this everyone has the same default settings for their storage. A lot of people are probably going to be looking for these default settings so to make it easy to find, here they are.
These are the AppSettings ones in the normal configuration file.
<appSettings>
<add key="AccountName" value="devstoreaccount1"/>
<add key="AccountKey" value="Eby8vdM02xNOcqFlqUwJPLlmEtlCDXJ1OUzFT50uSRZ6IFsuFq2UVErCz4I6tq/K1SZFPTOtr/KBHBeksoGMGw=="/>
<add key="BlobStorageEndpoint" value="http://localhost:10000"/>
<add key="QueueStorageEndpoint" value="http://localhost:10001"/>
<add key="TableStorageEndpoint" value="http://localhost:10002"/>
</appSettings>
These are the ones in the ServiceConfiguration.csfg file.
<ConfigurationSettings>
<Setting name="BlobStorageEndpoint" value="http://127.0.0.1:10000/" />
<Setting name="QueueStorageEndpoint" value="http://127.0.0.1:10001/" />
<Setting name="TableStorageEndpoint" value="http://127.0.0.1:10002/" />
<Setting name="AccountName" value="devstoreaccount1"/>
<Setting name="AccountSharedKey" value="Eby8vdM02xNOcqFlqUwJPLlmEtlCDXJ1OUzFT50uSRZ6IFsuFq2UVErCz4I6tq/K1SZFPTOtr/KBHBeksoGMGw=="/>
</ConfigurationSettings>
If you need access in both a WebRole and a WorkerRole this config section can be added to each one.
Also because you have added this to that configuration file you will need to add the definition of these to the ServiceDefinition.csdef file in the following way.
<ConfigurationSettings>
<Setting name="BlobStorageEndpoint"/>
<Setting name="QueueStorageEndpoint"/>
<Setting name="TableStorageEndpoint"/>
<Setting name="AccountName"/>
<Setting name="AccountSharedKey"/>
</ConfigurationSettings>
If you don't have this file matching the configuration you will get this nasty error message which doesn't tell you much. Thanks for telling me "Invalid configuration file." It doesn't say what file or what is wrong or anything.
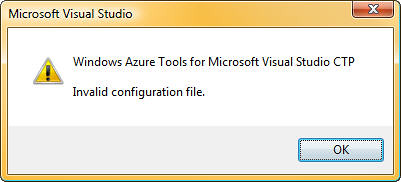
When you need to access Azure storage you will be using some of this information. Some helper methods have been created which access these values from your configuration. These will help you get StorageAccountInfo classes. You can use them in this way.
StorageAccountInfo queueAccount = StorageAccountInfo.GetDefaultQueueStorageAccountFromConfiguration();
StorageAccountInfo blobAccount = StorageAccountInfo.GetDefaultBlobStorageAccountFromConfiguration();
StorageAccountInfo tableAccount = StorageAccountInfo.GetDefaultTableStorageAccountFromConfiguration();
UPDATE: I forgot to mention when I first posted this that the Storage classes for this early release of Windows Azure are kept in one of the sample projects. It is the StorageClient sample project and the namespace needed is Microsoft.Samples.ServiceHosting.StorageClient. This will change once they've gotten some more substantial libraries set up for accessing Azure Storage.
This keeps things nice and clean, and makes connecting a lot easier.
Once you have these AccountInfo objects you can use them in the constructors for your service classes which give you access to the storage. You can access that storage using the following code.
QueueStorage queueService = QueueStorage.Create(queueAccount);
BlobStorage blobService = BlobStorage.Create(blobAccount);
TableStorage tableService = TableStorage.Create(tableAccount);
TableStorage tableService = TableStorage.CreateTablesFromModel(typeof(MyClass), tableAccount);
Please note that the table storage is slightly different from the other two. They are much easier since they don't vary in structure. I recommend you create that service based on a specific model class you have already defined. It should inherit from the TableStorageEntity class.
Now you're ready to start working with Azure storage.
Earlier this morning we saw some very interesting Keynote talks here. A preview of Windows 7 was shown. We're finally seeing some really great direction from Microsoft. I admit I am very much submerged in all that is Microsoft considering my occupation, but I have plenty of times in the past been one of the people to attack the mistakes of Microsoft. I think we've finally reached the stage in Internet integration that Microsoft was worried it would not be part of. Plenty of people say in the past that Microsoft got into the browser market because that is where everything was moving. Because of this they needed to be involved in this web-based revolution or they would miss everything.
In the past people would tell me that eventually it wouldn't matter what OS I ran, it would only matter what browser I used. This hasn't exactly happened, but it is moving that way slowly. I still think that operating systems are still EXTREMELY important. There certainly wouldn't be this PC versus Mac debate still raging if operating systems didn't matter. It is not all about the hardware here. PCs and Macs are not very different when it comes to hardware. They've got a few differences, but not much. The big difference is in the OS even today. Browser may work cross platform, but I am sure that web developers know that even looking at Firefox there are differences between Firefox on Windows and Firefox on Apple.
At the keynote Microsoft was very much pushing the integration they want to make between all devices. Client applications, web applications, and mobile applications all working together at the same time working with synchronized information. Allowing someone to leave one location continue working while in transit and continue that work once again at a new location on a new machine. This has been somewhat possible for a while, but not integrated well enough for it to be easy for people to do this regularly.
Windows Live Mesh was pushing some of this, but seemed not impressive enough to me as well as others. Now with the addition of Windows Azure there is a lot more which can be done. The advances made in Windows 7 as well as the new versions of Office coming can really make some differences.
I am certain I am not the only one who noticed the similarities between the web-based version of Microsoft Office and Google apps. I would say the big difference here is the integration they've made with the client versions of Office. Being the company which makes Office allows them to leverage the support needed to integrate so well that simultaneous work can be done on individual documents.
Developers, Developers, Developers.... I mean integration. If you haven't checked out the video of the keynote this morning I highly recommend watching it. You will get to see some great demonstrations of a lot of the new technology coming out of Redmond. I don't know if or where the video might be, but I will post it here if I find out. Since I was there I haven't bothered looking for the video.
I've used Python a decent amount in the past. I enjoy the language and I've taught the language to non-computer science students at Kent State University. There are plenty of things that I really love about that language. I could write plenty of blog posts regarding how totally awesome python is. I tend to use it for quick little bits of code. I don't use too much Python for production code, but I do like a lot of the language features.
One of the big pieces of Python I enjoy is the ability to define a default value for a parameter in a method. This allows for optional parameters. Wonderful stuff. I hate having to make extra overloads to handle default parameters which pass in either the right ones or values specifying that the value is missing. Way too much handling just to handle this case.
Named parameters are also a lot of fun since you're able to specify which parameter you're passing. It works pretty well in Python. I don't know how useful it will be in C#, but it is a neat feature which doesn't add much complexity to the language.
In the session I am sitting in at the PDC I am listening to a speaker talking about how this is coming down the pipe for C#. I would guess C# 4.0 will include these features. Now I can finally get rid of all of the extra overloads. I can finally just have my default parameters. No more of the extra overloads or passing in missing values.
For a lot of code we want to allow for customization, but at the same time we want to be able to have default values since the 90% case doesn't require the customization. We need the ability to customize some, so these features in combination could allow a method with say 8 parameters have you specify 2 of them and then name the 3rd one you want to specify. This should help clean up code and allow us to be concerned only with the code which is currently important to us. No need to worry about all the extra parameters in the method that we don't care about.
Finally!!! I've been wanting default and named parameters for a looooong time.
In the past the C# language obtained a new feature allowing it to declare variables as type "var". It didn't really do any dynamic typing though. For the most part the var is just there for developer convenience. Some people have complained about this in the past. Stating that C# 3.0 doesn't have dynamic types. The problem is that with that it is figuring out when compiling what type the variable is.
I am currently sitting in the Los Angeles Convention Center for the PDC 2008. I am watching a session on the future of C#. One of the interesting demos we're seeing in this is some of the new dynamic typing that is coming down the pipe. Variables are being defined with the type "dynamic" this is very cool because it is being figured out at runtime and not at compile time. It seems to be a very powerful ability of the language even in this early stage. Perhaps we'll see some new stuff from this some time soon.
Looks like they're combining the dynamic and var so they work very well together.
In the recent Keynote here at Microsoft PDC, they just announced what they're calling an operating system for the cloud. Windows Azure is a platform for developers to create applications which are highly scalable. Over a month ago I was at Microsoft's campus looking at their Red Dog project. Now we've all learned that Microsoft has named this project more officially as Windows Azure. With this new project they are launching their CTP solution. With this they have a live cloud on which people can publish their cloud-based applications.
For now hosting applications on the cloud will be free of charge for people. Once Microsoft moves further along with their cloud hosting abilities this will be a pay service. They've not said yet how much these services will cost or even really what you'll be charged for. It looks like there will be a lot of new Azure stuff coming out soon.
One of the neatest applications I've seen here is one that I saw a preview of when it was in its early development in Redmond. Bluehoo is a neat application. It is targeted to mobile users. It allows them to have their phones communicate with eachother and with central services hosted on the cloud. This allows all of the users to know who is around them and what those people's interests are based on profiles the users specify. There is so much potential for this application I can't wait to see what they do with it.
It would be great to be able to walk into an establishment of some kind and see that there are people sharing your common interests. You'll be able to start conversations with people based on this. According to the Bluehoo guys the application will be available as a beta service online later today. Go check it out.